Understanding Control Flow in Python: Loops and Conditional Statements
- programmersumu
- May 25, 2023
- 3 min read
Python is a versatile programming language that offers various control flow structures to handle the flow of execution within a program. Control flow refers to the order in which statements are executed based on certain conditions. Two fundamental components of control flow in Python are loops and conditional statements. In this blog, we will delve into the world of control flow in Python, exploring the different types of loops and conditional statements and understanding how they can be effectively used.
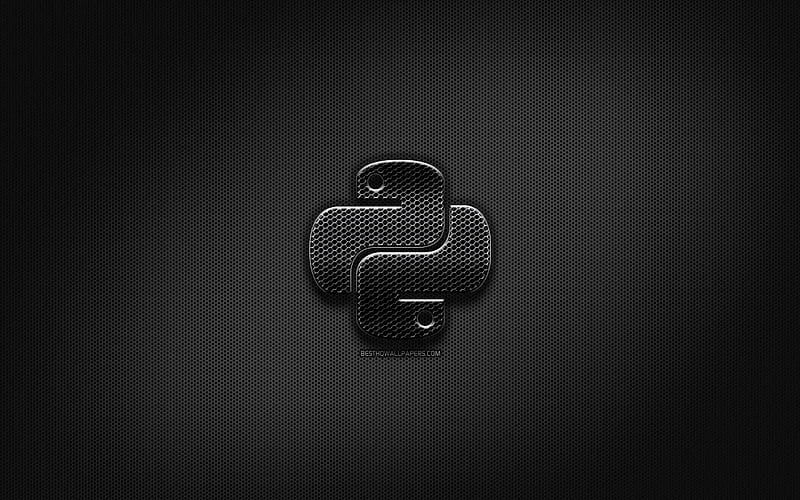
Loops in Python:
Loops are used to repeat a set of instructions or a block of code multiple times until a certain condition is met. Python provides two main types of loops: the "for" loop and the "while" loop.
1. The "for" Loop:
The "for" loop is used to iterate over a sequence of elements such as lists, tuples, or strings. It allows you to execute a block of code for each item in the sequence. Here's an example that demonstrates the usage of a "for" loop:
```
fruits = ["apple", "banana", "orange"]
for fruit in fruits:
print(fruit)
```
In this example, the loop iterates through the elements of the `fruits` list, and the `fruit` variable takes each value in the sequence one by one. The loop body, indicated by indentation, executes the `print` statement for each fruit in the list.
2. The "while" Loop:
The "while" loop repeats a block of code as long as a given condition is true. It is generally used when the number of iterations is unknown. Here's an example that demonstrates the usage of a "while" loop:
```
count = 0
while count < 5:
print("Count:", count)
count += 1
```
In this example, the loop will continue executing the indented code block until the `count` variable becomes equal to or exceeds 5. The `count` variable is incremented by 1 in each iteration to avoid an infinite loop.
Conditional Statements in Python:
Conditional statements allow us to execute different blocks of code based on specific conditions. Python provides three main types of conditional statements: "if," "elif," and "else."
1. The "if" Statement:
The "if" statement is used to perform a certain action or execute a block of code only if a particular condition is true. Here's an example that demonstrates the usage of an "if" statement:
```num = 10
if num > 0:
print("The number is positive.")
```
In this example, the code inside the "if" statement will execute only if the condition `num > 0` evaluates to true. Otherwise, the code block will be skipped.
2. The "elseif" Statement:
The "elseif" statement allows you to check for multiple conditions in sequence after the initial "if" statement. It is useful when you have more than one condition to evaluate. Here's an example that demonstrates the usage of an "elseif" statement:
```
num = 0
if num > 0:
print("The number is positive.")
elseif num < 0:
print("The number is negative.")
else:
print("The number is zero.")
```
In this example, the code will check the conditions one by one. If the first condition is false, it moves to the next "elif" statement, and if all conditions are false, the code inside the "else" block will execute.
3. The "else" Statement:
The "else" statement is used to execute a block of code when none of the preceding conditions in an "if" or "elif" statement
is true. It serves as the default option. Here's an example that demonstrates the usage of an "else" statement:
```
age = 17
if age >= 18:
print("You are eligible to vote.")
else:
print("You are not eligible to vote yet.")
```
In this example, if the condition `age >= 18` is false, the code inside the "else" block will execute, providing an alternative message.
Understanding control flow is essential for writing effective and functional Python programs. Loops and conditional statements provide the necessary tools to handle different scenarios by repeating code and making decisions based on conditions. With the knowledge gained from this blog, you can now confidently utilize loops and conditional statements in your Python programs, enhancing their functionality and flexibility. By mastering control flow, you unlock the power to create more dynamic and interactive applications. Happy coding!
Comentarios