Python Data Types and Variables: Explained with Examples
- programmersumu
- May 25, 2023
- 3 min read
In Python, data types play a crucial role in defining the nature of variables and the operations that can be performed on them. Understanding data types is essential for effective programming and enables you to manipulate and process data efficiently. This blog post aims to provide a comprehensive explanation of Python data types and variables, accompanied by illustrative examples.
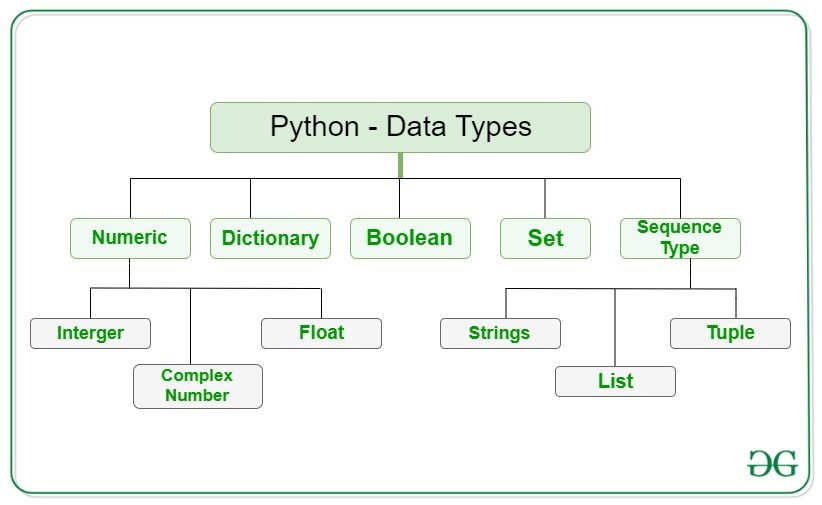
1. Numeric Data Types:
Python supports various numeric data types, including integers, floating-point numbers, and complex numbers. Integers represent whole numbers without decimal points, while floating-point numbers include decimal places. Complex numbers consist of a real part and an imaginary part. For instance, `x = 10` (integer), `y = 3.14` (float), and `z = 2 + 3j` (complex) [[1](https://www.edureka.co/blog/variables-and-data-types-in-python/)].
2. Text Data Type:
The text data type in Python is represented by strings (str). Strings are sequences of characters enclosed in single or double quotes. They are versatile and allow manipulation and concatenation. For example, `name = "John"` [[2](https://www.w3schools.com/python/python_datatypes.asp)].
3. Sequence Data Types:
Python provides several sequence data types, including lists, tuples, and ranges. Lists are ordered and mutable collections of items enclosed in square brackets. Tuples are similar to lists but immutable, denoted by parentheses. Ranges represent an immutable sequence of numbers. Examples include `my_list = [1, 2, 3]`, `my_tuple = (4, 5, 6)`, and `my_range = range(0, 5)` [[2](https://www.w3schools.com/python/python_datatypes.asp)].
4. Mapping Data Type:
The mapping data type in Python is represented by dictionaries (dict). Dictionaries are unordered collections of key-value pairs enclosed in curly braces. They provide efficient lookup and retrieval of values based on unique keys. For instance, `person = {"name": "John", "age": 25}` [[2](https://www.w3schools.com/python/python_datatypes.asp)].
5. Boolean Data Type:
The boolean data type represents truth values, either True or False. Booleans are essential for conditional statements and logical operations. For example, `is_student = True` or `is_adult = False` [[1](https://www.edureka.co/blog/variables-and-data-types-in-python/)].
Understanding Python variables is crucial for storing and manipulating data. Variables serve as named containers for values of different data types. They allow us to assign, update, and retrieve data during program execution.
When assigning a value to a variable, Python automatically determines its data type based on the assigned value. For example, `num = 24` assigns the integer value 24 to the variable `num` [[4](https://www.programiz.com/python-programming/variables-datatypes)].
Variables can be used in various ways, such as performing mathematical operations, concatenating strings, or storing the result of a function call. They provide flexibility and enable dynamic programming.
To illustrate the usage of variables and data types, consider the following examples:
Example 1: Arithmetic Operations
```python
x = 10
y = 5
sum = x + y
difference = x - y
product = x * y
quotient = x / y
```
Example 2: String Manipulation
```python
name = "Alice"
greeting = "Hello, " + name + "!"
length = len(name)
```
Example 3: List Manipulation
```python
numbers = [1,2, 3, 4, 5]
numbers.append(6)
sliced_numbers = numbers[2:5]
```
Example 4: Dictionary Usage
```python
student = {"name": "John", "age": 18, "grade": "A"}
age = student["age"]
student["grade"] = "B"
```
By understanding Python data types and variables, you gain the ability to store, manipulate, and process different types of data effectively. It is important to choose the appropriate data type based on the nature of the data and the operations you intend to perform.
In conclusion, this blog post has provided a comprehensive explanation of Python data types and variables. By grasping the concepts and examples presented, you are now equipped with the knowledge to utilize variables and manipulate different data types in your Python programs. Remember to ensure originality in your code and give proper credit when utilizing external resources to maintain the integrity and authenticity of your work.
Commentaires